Using the Ant make utility
|
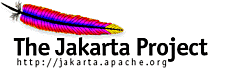
|
Introduction
|
|
I have started to use the Ant make utility that originates in the
Jakarta/Ant project for writing
make scripts. You can download the software from the Apache web site and install it on your
own machine. Make yourself familiar with the Ant utility.
An Ant script is a XML-based description of the project, its targets and tasks. It is easy to
understand, easy to use and maintain and has the advantage of a pure Java solution - that means you
can use it wherever you have a Java runtime environment.
|
|
Ant script to generate the DynaWorks API docs
|
|
Let's have a look at the first buildfile for an Ant project - the file
Docu.xml in the .\workspaces directory:
<!-- #################################################################
DynaWorks documentation
################################################################# -->
<project name="DynaWorks-Documentation"
default="makedoc"
basedir="g:/PalmDev/DynaWorks">
<!-- ================================================
set global path properties for this build
================================================ -->
<property name="SRC" value="${basedir}/src" />
<property name="DOCS" value="${basedir}/apidocs" />
<!-- ================================================
Task "PREPARE": create output directories.
================================================ -->
<target name="prepare">
<!-- ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Create the time stamp
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ -->
<tstamp />
<!-- ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Create the dir structure needed.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ -->
<mkdir dir="${DOCS}" />
</target>
<!-- ================================================
Task "MAKEDOC": create library documentation.
================================================ -->
<target name="makedoc" depends="prepare">
<javadoc sourcepath="${SRC}"
packagenames="brf.j2me.dynaworks.*"
destdir="${DOCS}"
overview="${SRC}/Overview.html"
private="true"
locale="en_US"
use="true"
version="true"
author="true"
Windowtitle="DynaWorks API Docs">
<classpath>
<pathelement path="f:\Java2\vame1.3\ive\lib\jclPalmXtr\classes.zip" />
<pathelement path="f:\Java2\ME\CLDC\bin\api\classes" />
</classpath>
</javadoc>
</target>
<!-- ================================================
Task "CLEAN": remove any files from previous builds.
================================================ -->
<target name="clean">
<!-- ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Delete the ${DOCS} directory tree.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ -->
<delete>
<fileset dir="${DOCS}" />
</delete>
<delete dir="${DOCS}/brf" />
</target>
</project>
|
You start the build process by issuing the following command and should get
something like the following output:
G:\PalmDev\DynaWorks\workspaces> ant -buildfile docu.xml
Buildfile: docu.xml
prepare:
makedoc:
[javadoc] Generating Javadoc
[javadoc] Javadoc execution
[javadoc] Loading source files for package brf.j2me.dynaworks...
[javadoc] Loading source files for package brf.j2me.dynaworks.db...
[javadoc] Loading source files for package brf.j2me.dynaworks.db.palm...
[javadoc] Loading source files for package brf.j2me.dynaworks.env...
[javadoc] Loading source files for package brf.j2me.dynaworks.env.j9...
[javadoc] Loading source files for package brf.j2me.dynaworks.env.kjava...
[javadoc] Loading source files for package brf.j2me.dynaworks.ui...
[javadoc] Loading source files for package brf.j2me.dynaworks.util...
[javadoc] Constructing Javadoc information...
[javadoc] Building tree for all the packages and classes...
[javadoc] Building index for all the packages and classes...
[javadoc] Building index for all classes...
[javadoc] Generating G:\PalmDev\DynaWorks\apidocs\stylesheet.css...
[javadoc] 0 error
BUILD SUCCESSFUL
Total time: 13 seconds
|
As you can see there are a some predefined tasks like calling the javadoc utility (or compiling
Java source code, packaging JAR files, creating and deleting directory and files and many, many more). Of course
you have to change the path definitions in an Ant script to match your own directory settings.
|
|
Extending Ant with new tasks
|
|
Although many tasks are predefined in Ant, there are always some tasks you need that are not
predefined. For example: In order to be able to use an Ant script for the DynaWorks/KJava
compilation, we need a task Preverify that preverifies all class files before they
can be used with the KJava/KVM implementation.
You can simply write your own Ant task in Java. The Ant documentation is quite explicit on that
topic, so I will not explain the whole process to detailed. First you include a new section into
the buildfile that gives your task a name and links it with an implementing class:
<!-- ================================================
describe user defined tasks.
================================================ -->
<taskdef name="Preverify" classname="brf.ant.tasks.PreverifyTask" />
|
The Java source for this task looks like this:
package brf.ant.tasks;
import java.io.*;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Task;
import org.apache.tools.ant.DirectoryScanner;
public class PreverifyTask extends Task {
// constructor.
public PreverifyTask () {
}
// The method executing the task
public void execute() throws BuildException {
// tell'em what's going on...
System.out.println (" [preverify] Processing files from '" + srcdir + "'");
System.out.println (" [preverify] Storing files to '" + destdir + "'");
// build base command string.
String optFile = srcdir + "/preverify.files";
String cmd = base + "/bin/preverify.exe @" + optFile;
// assemble the options file.
try {
PrintWriter opts = new PrintWriter (new BufferedWriter (new FileWriter (optFile)));
opts.println ("-classpath " + base + "/bin/api/classes;" + srcdir);
opts.println ("-d " + destdir);
DirectoryScanner ds = new DirectoryScanner ();
String[] incList = new String[] { includes };
ds.setIncludes (incList);
ds.setBasedir (new File (srcdir));
ds.scan();
String[] files = ds.getIncludedFiles ();
for (int i = 0; i < files.length;i++) {
// convert filename to class name
String line = files[i];
line = line.replace('\\', '.').substring (0, line.length() - 6);
opts.println (line);
}
opts.close ();
opts = null;
// execute
Runtime.getRuntime().exec(cmd).waitFor();
}
catch (Exception e) {
throw new BuildException (e.getMessage());
}
finally {
// remove options file.
new File (optFile).delete();
}
}
// The setter for the "base" attribute
public void setBase (String name) {
base = name;
}
// The setter for the "srcdir" attribute
public void setSrcdir (String name) {
srcdir = name;
}
// The setter for the "destdir" attribute
public void setDestdir (String name) {
destdir = name;
}
// The setter for the "includes" attribute
public void setIncludes (String name) {
includes = name;
}
// attributes.
private String base = null;
private String srcdir = null;
private String destdir = null;
private String includes = null;
}
|
|